Python Iterators Examples
Iterators are objects that can be used to iterate all the items in iterable objects such as Lists, Tuples, Dictionaries, and Sets. It means iterators have the capability to traverse through the iterable objects. A few Examples of iterators are enumerate, map, filter, zip, and reversed. Iterators are basically implemented for loops, comprehensions, and generators.
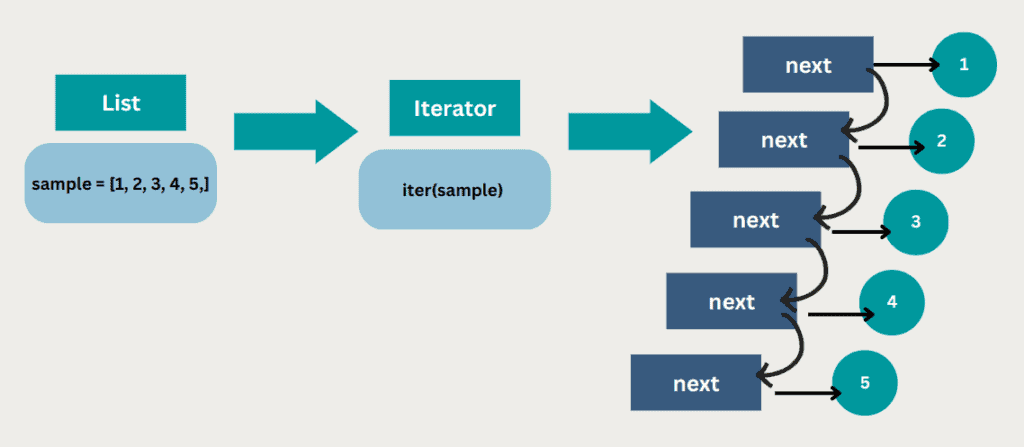
Let’s create an iterator and print the next item as shown in the above diagram:
# Create a list
sample = [1, 2, 3, 4, 5]
# Create an iterator
sample_iter = iter(sample)
# Print the next item
print(next(sample_iter))
print(next(sample_iter))
print(next(sample_iter))
print(next(sample_iter))
print(next(sample_iter))
Output: 1 2 3 4 5
Create Your own Iterator
In Python, iterators can be created using an iterator object. In order to implement an iterator should have two methods __iter__() and __next__().
- __iter__(): returns the iterator object itself.
- __next__(): returns the next value in the sequence.
in order to avoid the iteration going on forever, raise the StopIteration Exception.
# Define class
class sample_iterator:
def __init__(self, n):
self.i = 0
self.n = n
def __iter__(self):
return self
def __next__(self):
if self.i < self.n:
i = self.i
self.i += 1
return self.i
else:
raise StopIteration
# Create Object
iter_obj = sample_iterator(9)
print(next(iter_obj))
print(next(iter_obj))
print(next(iter_obj))
print(next(iter_obj))
Output: 1 2 3 4
Let’s convert the iterator object into a list iterable:
iter_obj = sample_iterator(9)
print(list(iter_obj))
Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Let’s use the iterator object in For loop:
iter_obj = sample_iterator(9)
for i in iter_obj:
print(i, end = " ")
iter_obj = sample_iterator(9)
while True:
try:
x = iter_obj.__next__()
print(x, end = " ")
except StopIteration:
break
Output: 1 2 3 4 5 6 7 8 9