Solving Blending Problem in Python using Gurobi
Learn how to use Python Gurobi to solve the blending problems using Linear Programming.
A blending problem is very similar to a diet problem. It is a well-known optimization problem. The main objective in such type of problem is to find the optimum combination of mixing different intermediates items or ingredients in order to minimize blending cost, meeting the quality standards and demand requirements of the final product.
The blending problem has have been used in various different applications and industries coal blending, blending of chemical fertilizers, mineral blending, Food processing. In the food processing industry, it is used to blend the number of ingredients with minimum cost. For example, multi-grain bread requires the blending of grains. blending tea recipe, blending juice or energy drinks.
In this tutorial, we will solve the blending problem for a food processing company. In the blending problem, we find a mix of ingredients for developing any food item. For example, A food processing manager wants to develop a new formula for Juice with a given restriction of sugar, levels of sugar in fruits, and cost of fruits while still meeting their nutritional standards. In such a problem, the manager can formulate the LP problem.
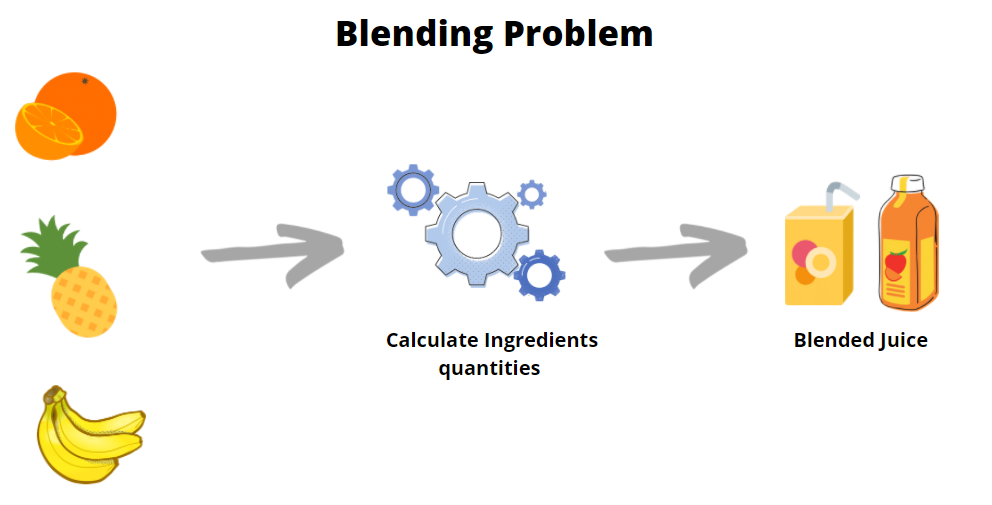
In this tutorial, we are going to cover the following topics:
Modeling Linear Programming Using Python Gurobi
There are various excellent optimization python packages are available such as SciPy, PuLP, Gurobi, and CPLEX. In this article, we will focus on the gurobipy python library.
Install gurobipy package:
!pip install gurobipy
GurobiPy modeling process has similar solving steps just like the PuLP library. Here are the steps for solving LP problems using gurobipy:
- Initialize Model
- Define Decision Variable
- Define Objective Function
- Define the Constraints
- Solve Model
Initialize Blending Problem Model
In this step, we will import all the classes and functions of gurobipy module and create a new model object using Model class.
# Import gurobipy library
from gurobipy import *
# Create an empty new model
model = Model()
Define Decision Variable
In this step, we will define the decision variables. In our problem, we have two variables ingredient-1 and ingredient-2. Let’s create them using addVar()
method. The addVar()
will take the following two values:
- First is the type of data (Continuous, Integer, Binary). Here,
'C'
for continuous,'B'
for binary,'I'
for integer,'S'
for semi-continuous, or'N'
for semi-integer. - Second is the name of whatever the variable represent.
Binary variables must be either 0 or 1. Integer variables can take any integer value between the specified lower and upper bounds. Semi-continuous variables can take any value between the specified lower and upper bounds, or a value of zero. Semi-integer variables can take any integer value between the specified lower and upper bounds, or a value of zero.
Let’s first create decision variables using addvar() method:
# Define Decision Variables
x1 = model.addVar(vtype="C", name="x1")
x2 = model.addVar(vtype="C", name="x2")
Define Objective Function
In this step, we will define the minimum objective function by adding it to the Model
object using setObjective()
method.
- expr: objective function expression. Argument can be a linear or quadratic expression.
- sense (optional): Optimization sense (GRB.MINIMIZE for minimization, GRB.MAXIMIZE for maximization).
# Define objective function
model.setObjective(4*x1 + 5*x2 , GRB.MINIMIZE)
Define the Constraints
Constraint captures the restriction on the values of the decision variables. The simplest example is a linear constraint, which states that a linear expression on a set of variables takes a value that is either less-than-or-equal, greater-than-or-equal, or equal to another linear expression.
Here, we are adding two types of constraints: Protein constraints and Carbohydrates constraints. We are also adding the non-negativity constraints.
# Define constraints
model.addConstr(3*x1 + 5*x2 >= 30, "c1") # Protein in grams
model.addConstr(3*x1 + 2*x2 >= 24, "c2") # Carbohydrates in grams
model.addConstr(x1 >= 0, "c3")
model.addConstr(x2 >= 0, "c4")
Solve Model
In this step, we will solve the LP problem by calling optimize() method. We can print the final value by using the following for loop.
# Run the optimization
model.optimize()
for v in model.getVars():
print(v.varName, v.x)
# The optimised objective function value
print('Minimized profit:', model.objVal)
Output: x1 6.666666666666667 x2 2.0 Minimized profit: 36.66666666666667Objective Function = 5.0
From the above results, we can infer that the optimal amounts of the two ingredients are 6.67 and 2.0.
Summary
In this article, we have learned about Blending Problem, Problem Formulation, and implementation using the python gurobipy library. We have solved the Blending Problem using a Linear programming problem in Python. Of course, this is just a simple case study, we can add more constraints to it and make it more complicated. In upcoming articles, we will write more on different optimization problems such as transshipment problem, assignment problem, balanced diet problem. You can revise the basics of mathematical concepts in this article and learn about Linear Programming using PuLP in this article.