Solving Balanced Diet Problem in Python using PuLP
Learn how to use Python PuLP to solve the Balanced Diet Problem using Linear Programming.
A proper diet is essential for each and every human being. It keeps them healthy, fit, and more prone to chronic diseases. Junk foods may cause severe health issues in the modern world. Achieving a balanced diet is a multi-variate optimization problem. It comprises various variables that need to be optimized at less cost.
The linear programming method can be considered as one of the ideal methods for optimizing the diet problem. Linear programming is one of the operation research tools for the decision-making process. Linear programming methods may reduce the cost of business problems. The main goal of the Balanced Diet Problem is used to optimize the nutritional need of the people at minimum cost using Linear programming.
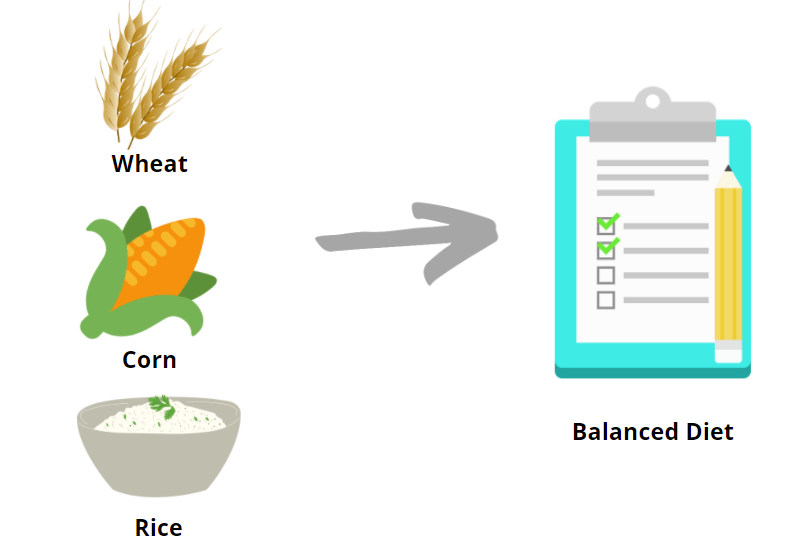
In this tutorial, we are going to cover the following topics:
Initialize Balanced Diet Problem Model
Let’s see the balanced diet problem example available in Book Introduction to Management Science.
In this problem, we need to design the composition of food items wheat, rice, and corn flakes in a 12-ounce cereal box.
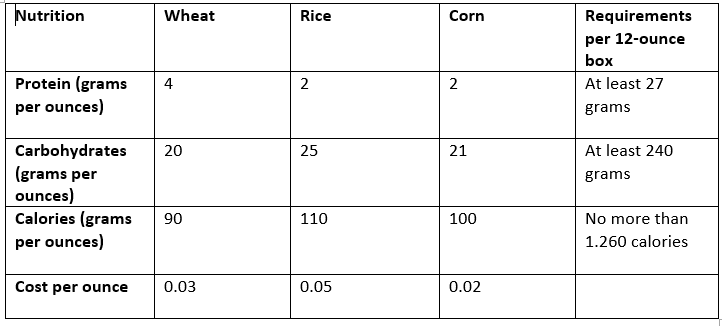
Let’s determine the optimal quantities of wheat, rice, and corn per box.
In this step, we will import all the classes and functions of pulp
module and create a Minimization LP problem using LpProblem class.
# Import all classes of PuLP module
from pulp import *
# Create the problem variable to contain the problem data
model = LpProblem("Balanced Diet Problem", LpMinimize)
Define Decision Variable
In this step, we will define the decision variables. In our problem, we have three variables wood tables, chairs, and bookcases. Let’s create them using LpVariable
class. LpVariable
will take the following four values:
- First, arbitrary name of what this variable represents.
- Second is the lower bound on this variable.
- Third is the upper bound.
- Fourth is essentially the type of data (discrete or continuous). The options for the fourth parameter are
LpContinuous
orLpInteger
.
# Define Decision Variables
x1 = LpVariable("wheat", 0, None, LpContinuous)
x2 = LpVariable("rice", 0, None, LpContinuous)
x3 = LpVariable("corn", 0, None, LpContinuous)
Define Objective Function
In this step, we will define the minimum objective function by adding it to the LpProblem
object.
# Define Objective
model += 0.03 * x1 + 0.05 * x2 + 0.02 * x3
Define the Constraints
Constraint captures the restriction on the values of the decision variables. The simplest example is a linear constraint, which states that a linear expression on a set of variables takes a value that is either less-than-or-equal, greater-than-or-equal, or equal to another linear expression.
In this step, we will add the 4 constraints defined in the problem by adding them to the LpProblem
object.
# Define Constraints
model += 4*x1 + 2*x2 + 2*x3 >= 27 # Protien (grams per ounce)
model += 420*x1 + 25*x2 + 21*x3 >= 240 # Carbohyderate (grams per ounce)
model += 90*x1 + 110*x2 + 100*x3 >= 27 # Calories (grams per ounce)
model += x1 + x2 + x3 >= 12 # Box Size 12 Ounce
Solve Model
In this step, we will solve the LP problem by calling solve() method. We can print the final value by using the following for loop.
# The problem is solved using PuLP's choice of Solver
model.solve()
# Print the variables optimized value
for v in model.variables():
print(v.name, "=", v.varValue)
# The optimised objective function value is printed to the screen
print("Value of Objective Function = ", value(model.objective))
Output: corn = 10.5 rice = 0.0 wheat = 1.5 Value of Objective Function = 0.255
From the above results, we can infer that the optimal amount of three food items is 10.5, 0.0, and 1.5 ounces of food item per box.
Summary
In this article, we have learned about Balanced Diet problems, Problem Formulation, and implementation using the python pulp
library. We have solved the Balanced Diet Problem example using a Linear programming problem in Python. Of course, this is just a simple case study, we can add more constraints to it and make it more complicated. In upcoming articles, we will write more on different optimization problems such as multiperiod production, network flow problems. You can revise the basics of mathematical concepts in this article and learn about Linear Programming using PuLP in this article. I have written more articles on different optimization problems such as transshipment problems, assignment problems, blending problems.